Optimizing Next.js SEO: A Comprehensive Guide to Metadata and Web Shareability
KACEY
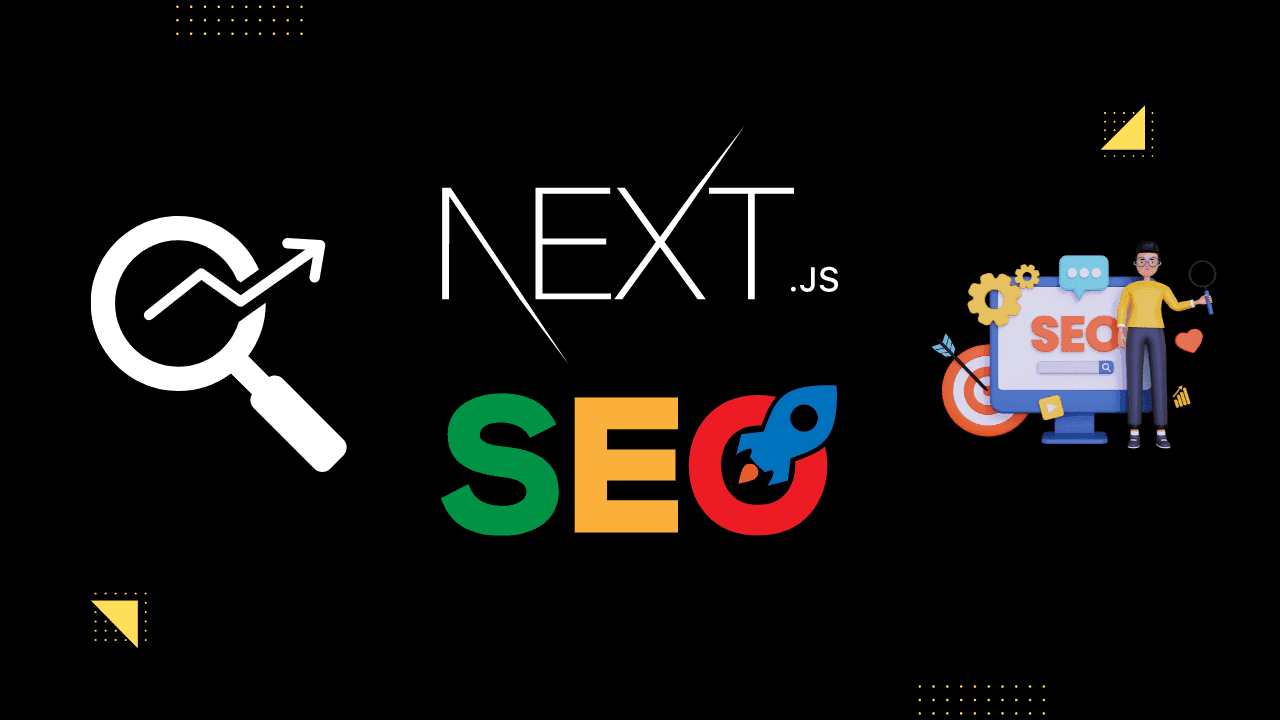
In this guide, we'll walk through the process of creating a Next.js application and adding metadata for SEO and web shareability. Metadata plays a crucial role in improving your site's visibility on search engines and enhancing how it appears when shared on social media platforms.
Before we begin, make sure you have Node.js and npm (Node Package Manager) installed on your machine.
Let's start by setting up a new Next.js project. If you haven't already, you can create a new Next.js application using the following commands:
npx create-next-app my-next-app
cd my-next-app
npm install
This will create a new Next.js project in a directory called my-next-app.
To add static metadata to your Next.js application, export a Metadata object from a layout.js or a static page.js file. Here's an example:
// pages/index.js
import { Metadata } from 'next'
export const metadata = {
title: 'My Next.js App',
description: 'A sample Next.js application with metadata.',
}
export default function Home() {
return (
// Your homepage content goes here
)
}
In this example, we've defined the title and description for our homepage.
For metadata that requires dynamic values, you can use the generateMetadata function. This function allows you to fetch data and construct metadata based on the current context. Here's an example:
// pages/products/[id].js
import { Metadata } from 'next'
// Define your dynamic metadata generation function
export async function generateMetadata({ params }) {
const id = params.id
const product = await fetch(`https://api.example.com/products/${id}`).then((res) => res.json())
// Optionally extend parent metadata
const previousImages = (await parent).openGraph?.images || []
return {
title: product.name,
openGraph: {
images: [`/images/${product.id}.jpg`, ...previousImages],
},
}
}
export default function Product({ product }) {
return (
// Your product page content goes here
)
}
In this example, we're dynamically generating metadata for a product page.
Next.js also allows you to define metadata using special files. These files include favicon.ico, apple-icon.jpg, icon.jpg, robots.txt, and sitemap.xml. You can use these files for static metadata or generate them programmatically with code.
Remember that file-based metadata has a higher priority and will override config-based metadata.
In this guide, we've learned how to set up a Next.js application and add metadata for SEO and web shareability. Whether you need static or dynamic metadata, Next.js provides flexible options to improve your site's visibility and shareability on the web.
For more details and advanced usage, refer to the Next.js Metadata API Reference.